Golang REST API Starter Kit
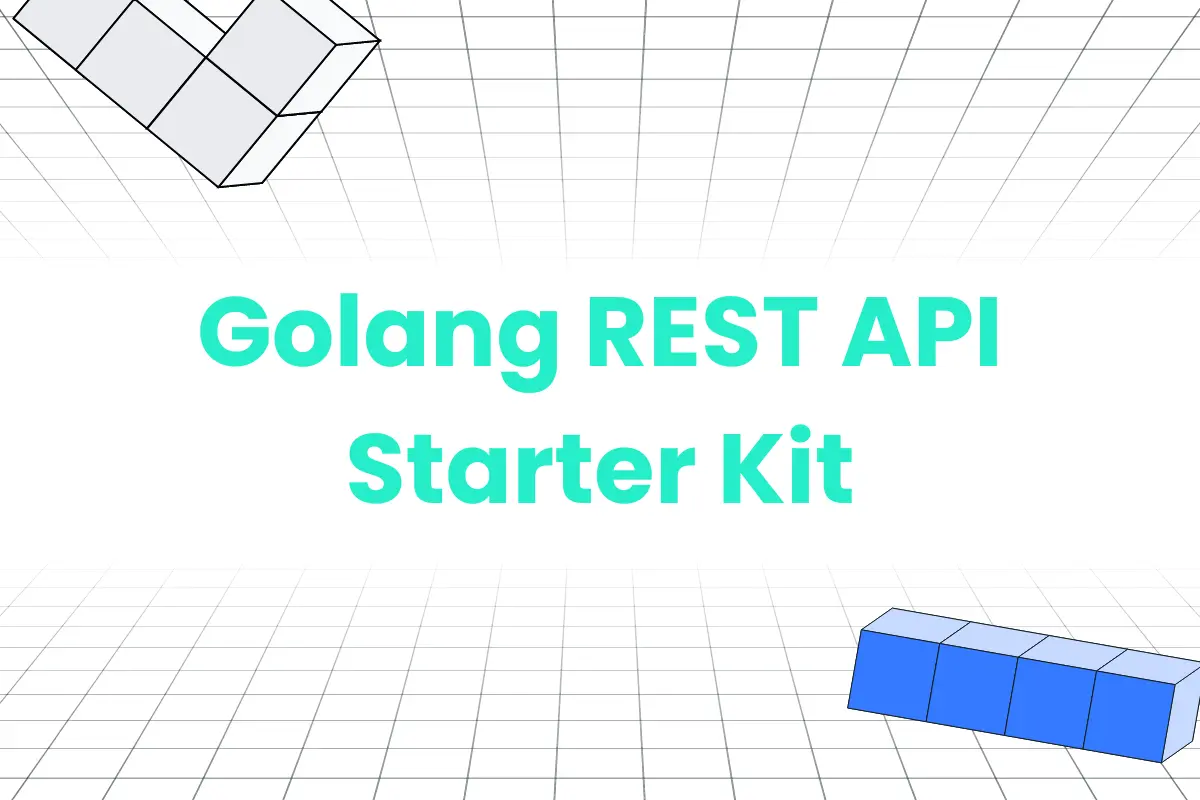
Table of contents
Are you looking for a Golang rest API starter kit to get your project off the ground? Look no further! This Golang rest API starter kit has everything you need to get started, from sample code to best practices. It's easy to set up and customize to create the perfect foundation for your project. With the Golang rest API starter kit, you'll be able to create powerful applications quickly and easily.
Why use Golang for your next REST API?
Go, also known as Golang, is an open-source programming language developed by Google. Go was designed for large-scale projects and can scale easily. Go offers a wide range of libraries and packages which provide useful functions and features such as authentication, routing, logging, and testing. Finally, Go’s concurrency model is designed to handle multiple requests simultaneously while avoiding resource contention. This makes it well-suited for building high-performance, high-traffic APIs that can handle heavy loads.
REST API is an architectural style that enables clients to access and manipulate web resources using HTTP protocol. The API defines a set of standard HTTP methods, such as GET, POST, PUT, DELETE, HEAD, OPTIONS, and PATCH. The REST API typically provides a uniform interface for a wide range of clients, including mobile devices, browsers, and servers. A well-designed REST API should be intuitive, flexible, secure, and easy to scale. With the help of Golang, you can create a robust REST API with all these qualities.
- Go is a great choice for developing a REST API since it provides excellent performance, scalability, and security.
- Additionally, servers built with Go experience instant start up times and are cheaper to run in pay-as-you-go and serverless deployments.
- And, to top it all off, Kubernetes and Docker - two of the most popular container orchestration platforms - are built using Go.
Step 1: Setting up the development environment
For anyone looking to build a robust and reliable rest API with Golang, the first step is to get your development environment up and running.
1. Installing Go on your computer
- The official download page for Go provides binary distributions for Mac OS, Windows, and Linux platforms.
- Depending on the operating system, you’ll need to select the appropriate file and follow the installation instructions.
- After installation, verify that it has been installed properly by running “go version” in your terminal.
2. Setting up a project directory and configuring Go workspace
Before you start coding, you need to set up a project directory to contain your source code files.
- To do this, open a terminal window and enter the command “go mod init <project-name>”. This will create a new folder within your current working directory with the name of your project.
- To configure your workspace, you’ll need to set the GOPATH environment variable to point to your project folder.
3. Installing necessary dependencies and tools
Now that your environment is set up, you can begin installing the necessary dependencies and tools.
- For example, if you’re using Gorilla Mux or Gin for routing, you’ll need to install them via the command “go get <package-name>.”
- Additionally, if you’re following the Golang clean architecture rest API approach, you may want to use a golang rest API scaffolding tool such as Splinter or Gorton to help structure your codebase.
- Finally, if you’re starting from scratch, use frameworks such as Echo to simplify the process of creating a golang project structure rest API.
Step 2: Installing the dependencies
When it comes to creating a REST API in Golang, there are many options available. From using an existing Go starter kit to building your own from scratch, it all depends on what you need and how much time you want to invest.
If you’re looking for a quick and easy way to get started with a Golang REST API, then the Golang Clean Architecture Rest API is just the right tool for you. This framework allows you to easily set up your project and install all the necessary dependencies with just a few clicks.
- The first step is downloading the framework's latest version from GitHub. Once you’ve done that, you can start installing the necessary packages. These include the Gin web framework, Gorm ORM, and more. Additionally, if you’re looking for extra features such as authentication or validation, you can also add those in.
- Once you’ve installed all the required packages, you’ll have a fully functioning Go starter kit. With this go-starter kit, you can create a fully functional rest api application with ease. You will have access to the full range of features provided by the framework such as routing, controllers, models, and views.
The Golang Clean Architecture Rest API provides an excellent foundation for creating a robust REST API application quickly and easily. By following the steps above, you can have your own Go REST API up and running in no time.
Step 3: Building the Rest API
1. Defining routes and handlers
Building a Rest API with Golang is a great way to quickly start creating web applications and services. One popular library for building web services with Golang is Gorilla Mux. It provides a powerful routing system and many other features. Another great choice is the GoFiber framework, which makes it easy to build lightweight, fast, secure web services. Finally, there’s Gin/Gonic, an HTTP framework for Go which is also popular for creating APIs.
When setting up a project, it’s important to follow a golang project structure. This means having a clear layout for files, folders, and packages, which helps keep your project organized. For Rest APIs in particular, a good structure includes separating the application components into ‘handlers’ and ‘models’. Handlers are responsible for handling the requests made to the API while models contain the data. This separation allows you to create modular code that can be easily maintained and extended.
2. Implementing CRUD operations using a database
To implement CRUD operations using a database such as MongoDB, MySQL, and PostgreSQL, with cloud solutions from AWZ, Azure, Google Cloud, etc., we need to include the relevant packages in our Go project. Depending on the type of database used, the packages will differ. For example, if using MongoDB as the database, we would need to include mgo/bson for basic object-document mapping. If using MySQL as the database, we would need to include MySQL package for driver connectivity and query building.
Once we have included the relevant packages for our database, we can start implementing CRUD operations for our Rest API. The CRUD operations could be handled with an ORM package such as gorm, which provides simple functions for creating, reading, updating, and deleting records in a database. Gorm also allows us to access different databases through its adapters.
3. Validating user input and handling errors
When building a Rest API, validating user input and handling errors is an essential part of the development process. Without proper validation, malicious users can take advantage of security vulnerabilities and damage the reliability of your application. To protect your REST API from malicious attacks and data loss, it is important to have an effective validation and error-handling strategy in place.
Various libraries and frameworks are available to help you implement such strategies in your project. A popular choice for handling user input and errors is Gorilla Mux, but since it is no longer maintained, you may want to consider using other libraries and frameworks, such as GoFiber or Gin Gonic. With the use of these libraries and frameworks, you can add robust validation rules and create custom error messages. Furthermore, these libraries also make it easy to add authorization checks, rate limiters, and other security measures.
Using libraries like GoFiber or Gin Gonic can make it easier for you to implement robust validation rules and custom error messages for your API. Furthermore, using Golang's project structure will help you keep your codebase organized and maintainable.
4. Implementing authentication and authorization
When setting up a Golang REST API, it is important to implement authentication and authorization to ensure the API is secure.
Note: Authentication is the process of verifying a user's identity, while authorization is the process of ensuring that a user has access to specific data or actions.
Several libraries are available for use in a Golang project structure to handle authentication and authorization. A popular one used to be Gorilla Mux; however, it is no longer maintained. GoFiber is a good alternative, and Gin/Gonic is another popular HTTP framework for Go. For even faster development, you can also use a Golang starter kit like GoStack, which contains all of the necessary components for setting up a secure REST API.
With the right libraries and tools in place, you can easily implement authentication and authorization for your Golang REST API. From using industry-standard cryptographic algorithms such as HMAC, JWT, and BCrypt to implementing various authentication methods like basic authentication, OAuth 2.0, and session authentication, you can ensure that your users are accessing a secure REST API. Similarly, authorization methods like role-based access control (RBAC), claims-based authorization, and policy-based authorization can also be implemented to ensure users only have access to the resources they are authorized for.
Step 4: Testing the API
When developing a Golang project, it is essential to have robust test coverage. Testing the REST API is no different. The process of testing a REST API can be broken down into two parts: setting up a test environment and writing unit and integration tests.
1. Setting up a Test Environment and Dependencies:
First, you must set up a test environment and install dependencies. This includes setting up tools such as Postman, a powerful tool for testing REST APIs. Other libraries such as mocks and assertions, are also important for writing comprehensive tests.
2. Writing Unit and Integration Tests:
Once the test environment is set up, you can start writing unit and integration tests for your Go Starter Kit Rest API. It is important to use TDD (Test Driven Development) methodology for writing these tests.
3. Using Tools Such as Postman to Test the API Endpoints:
When it comes to testing the API endpoints, Postman can be very useful. With Postman, you can easily create, send and review HTTP requests. You can also use the Postman collections feature to store the requests to use them in automated integration tests.
Testing a REST API requires time, effort, and knowledge of best practices. Using the right tools and having good test coverage is essential for ensuring that your Go Starter Kit Rest API works as expected.
The project structure also makes it easy to mock and inject the dependencies needed for testing, allowing you to focus on writing your tests instead of setting up complex fixtures. Additionally, there is an example Golang REST-API example on GitHub that you can use as a reference to ensure your code meets best practices.
Step 5: Deploying the API
Once your Golang REST API is ready to be deployed, you will need to consider a few things. Depending on your application, there may be specific requirements for deploying the API. Regardless of your specific requirements, there are a few steps to keep in mind when deploying a Golang REST API.
- If you are using a Go Starter Kit such as the golang clean architecture rest api or go starter kit available on GitHub, you can leverage the provided example code and configuration files to streamline the process. This step can save time and effort and make the process of deploying the API much simpler.
- It is important to consider the hosting environment when deploying your API. If you are using a cloud-based platform, such as Amazon Web Services or Google Cloud Platform, then you should use their provided tools and services to ensure that your API is securely hosted and able to scale as needed.
- After your API is up and running, you should ensure that all endpoints are working correctly and that security measures are in place. You may also want to consider testing your API with a service such as Postman or Insomnia to ensure it meets your application's requirements.
Once all of these steps are complete, your Golang REST API will be ready for use! By following these guidelines, you can ensure that your API is secure and reliable for use in production applications.
Step 6: Logging
Logging is a crucial component of any Golang project structure, especially when it comes to Rest APIs. Logging helps developers keep track of errors and other activities during development and debugging. For instance, by logging errors, developers can identify and address problems quickly.
Using a reliable logging solution can make the process of debugging a Go starter kit easier. Common logging solutions include Datadog, Sentry, and Elasticsearch. With Datadog, developers can quickly diagnose and debug issues in their Rest API applications. Sentry provides a powerful monitoring system to help catch errors and send notifications to developers. Finally, Elasticsearch enables developers to easily store, search, and analyze logs.
Using a reliable logging solution in your Golang Rest API starter kit can improve your applications' overall performance.
Suggested Read: Golang vs Java for Microservices
Conclusion
The Golang Rest API starter kit is a great way to quickly start your own API project. It comes with all the necessary components to swiftly scaffold a rest API, including setting up a database, creating handlers, and testing the API. With the help of the Golang Rest API starter kit, you can create a robust and efficient API in no time.
FAQs
1. How do I get started with creating a REST API in Go?
Once Golang is set up, it’s time to create your workspace. Next, you will need to write the code for your first endpoint. Once you’ve written your code, it’s time to test it out. You can use a tool such as Postman to send requests to your API endpoints and ensure they function correctly. Once you’ve tested your first endpoint, you can move on to adding more endpoints.
2. How do I structure my Go code for a REST API?
By separating the components into their own packages, you can easily identify where any changes need to be made and make sure they don't affect other parts of the application.
Also, it's important to use best practices such as using comments and documentation where appropriate, following standards for indentation, and separating business logic from view logic. This will help you find any potential errors or areas that need improvement.
3. How do I handle database interactions in my Go REST API?
While the native ‘database/SQL package is great for developers who are comfortable with SQL, those who are not may prefer an ORM library like GORM or working with a NoSQL database such as MongoDB or Redis. Whichever route you take, ensuring that your code is well-structured and tested will be key to creating a robust and secure REST API in Go.
4. How do I handle user authentication and authorization in my Go REST API?
The first option is to use a third-party library such as go-auth or oauth2. These libraries offer comprehensive solutions for user authentication and authorization. The second option is to build your own authentication system from scratch. This allows you to customize the system for your particular needs. To do this, you'll need to implement a session store, a way to encrypt passwords, and a way to limit access to certain resources.
Are you looking for a Golang rest API starter kit to get your project off the ground? Look no further! This Golang rest API starter kit has everything you need to get started, from sample code to best practices. It's easy to set up and customize to create the perfect foundation for your project. With the Golang rest API starter kit, you'll be able to create powerful applications quickly and easily.
Why use Golang for your next REST API?
Go, also known as Golang, is an open-source programming language developed by Google. Go was designed for large-scale projects and can scale easily. Go offers a wide range of libraries and packages which provide useful functions and features such as authentication, routing, logging, and testing. Finally, Go’s concurrency model is designed to handle multiple requests simultaneously while avoiding resource contention. This makes it well-suited for building high-performance, high-traffic APIs that can handle heavy loads.
REST API is an architectural style that enables clients to access and manipulate web resources using HTTP protocol. The API defines a set of standard HTTP methods, such as GET, POST, PUT, DELETE, HEAD, OPTIONS, and PATCH. The REST API typically provides a uniform interface for a wide range of clients, including mobile devices, browsers, and servers. A well-designed REST API should be intuitive, flexible, secure, and easy to scale. With the help of Golang, you can create a robust REST API with all these qualities.
- Go is a great choice for developing a REST API since it provides excellent performance, scalability, and security.
- Additionally, servers built with Go experience instant start up times and are cheaper to run in pay-as-you-go and serverless deployments.
- And, to top it all off, Kubernetes and Docker - two of the most popular container orchestration platforms - are built using Go.
Step 1: Setting up the development environment
For anyone looking to build a robust and reliable rest API with Golang, the first step is to get your development environment up and running.
1. Installing Go on your computer
- The official download page for Go provides binary distributions for Mac OS, Windows, and Linux platforms.
- Depending on the operating system, you’ll need to select the appropriate file and follow the installation instructions.
- After installation, verify that it has been installed properly by running “go version” in your terminal.
2. Setting up a project directory and configuring Go workspace
Before you start coding, you need to set up a project directory to contain your source code files.
- To do this, open a terminal window and enter the command “go mod init <project-name>”. This will create a new folder within your current working directory with the name of your project.
- To configure your workspace, you’ll need to set the GOPATH environment variable to point to your project folder.
3. Installing necessary dependencies and tools
Now that your environment is set up, you can begin installing the necessary dependencies and tools.
- For example, if you’re using Gorilla Mux or Gin for routing, you’ll need to install them via the command “go get <package-name>.”
- Additionally, if you’re following the Golang clean architecture rest API approach, you may want to use a golang rest API scaffolding tool such as Splinter or Gorton to help structure your codebase.
- Finally, if you’re starting from scratch, use frameworks such as Echo to simplify the process of creating a golang project structure rest API.
Step 2: Installing the dependencies
When it comes to creating a REST API in Golang, there are many options available. From using an existing Go starter kit to building your own from scratch, it all depends on what you need and how much time you want to invest.
If you’re looking for a quick and easy way to get started with a Golang REST API, then the Golang Clean Architecture Rest API is just the right tool for you. This framework allows you to easily set up your project and install all the necessary dependencies with just a few clicks.
- The first step is downloading the framework's latest version from GitHub. Once you’ve done that, you can start installing the necessary packages. These include the Gin web framework, Gorm ORM, and more. Additionally, if you’re looking for extra features such as authentication or validation, you can also add those in.
- Once you’ve installed all the required packages, you’ll have a fully functioning Go starter kit. With this go-starter kit, you can create a fully functional rest api application with ease. You will have access to the full range of features provided by the framework such as routing, controllers, models, and views.
The Golang Clean Architecture Rest API provides an excellent foundation for creating a robust REST API application quickly and easily. By following the steps above, you can have your own Go REST API up and running in no time.
Step 3: Building the Rest API
1. Defining routes and handlers
Building a Rest API with Golang is a great way to quickly start creating web applications and services. One popular library for building web services with Golang is Gorilla Mux. It provides a powerful routing system and many other features. Another great choice is the GoFiber framework, which makes it easy to build lightweight, fast, secure web services. Finally, there’s Gin/Gonic, an HTTP framework for Go which is also popular for creating APIs.
When setting up a project, it’s important to follow a golang project structure. This means having a clear layout for files, folders, and packages, which helps keep your project organized. For Rest APIs in particular, a good structure includes separating the application components into ‘handlers’ and ‘models’. Handlers are responsible for handling the requests made to the API while models contain the data. This separation allows you to create modular code that can be easily maintained and extended.
2. Implementing CRUD operations using a database
To implement CRUD operations using a database such as MongoDB, MySQL, and PostgreSQL, with cloud solutions from AWZ, Azure, Google Cloud, etc., we need to include the relevant packages in our Go project. Depending on the type of database used, the packages will differ. For example, if using MongoDB as the database, we would need to include mgo/bson for basic object-document mapping. If using MySQL as the database, we would need to include MySQL package for driver connectivity and query building.
Once we have included the relevant packages for our database, we can start implementing CRUD operations for our Rest API. The CRUD operations could be handled with an ORM package such as gorm, which provides simple functions for creating, reading, updating, and deleting records in a database. Gorm also allows us to access different databases through its adapters.
3. Validating user input and handling errors
When building a Rest API, validating user input and handling errors is an essential part of the development process. Without proper validation, malicious users can take advantage of security vulnerabilities and damage the reliability of your application. To protect your REST API from malicious attacks and data loss, it is important to have an effective validation and error-handling strategy in place.
Various libraries and frameworks are available to help you implement such strategies in your project. A popular choice for handling user input and errors is Gorilla Mux, but since it is no longer maintained, you may want to consider using other libraries and frameworks, such as GoFiber or Gin Gonic. With the use of these libraries and frameworks, you can add robust validation rules and create custom error messages. Furthermore, these libraries also make it easy to add authorization checks, rate limiters, and other security measures.
Using libraries like GoFiber or Gin Gonic can make it easier for you to implement robust validation rules and custom error messages for your API. Furthermore, using Golang's project structure will help you keep your codebase organized and maintainable.
4. Implementing authentication and authorization
When setting up a Golang REST API, it is important to implement authentication and authorization to ensure the API is secure.
Note: Authentication is the process of verifying a user's identity, while authorization is the process of ensuring that a user has access to specific data or actions.
Several libraries are available for use in a Golang project structure to handle authentication and authorization. A popular one used to be Gorilla Mux; however, it is no longer maintained. GoFiber is a good alternative, and Gin/Gonic is another popular HTTP framework for Go. For even faster development, you can also use a Golang starter kit like GoStack, which contains all of the necessary components for setting up a secure REST API.
With the right libraries and tools in place, you can easily implement authentication and authorization for your Golang REST API. From using industry-standard cryptographic algorithms such as HMAC, JWT, and BCrypt to implementing various authentication methods like basic authentication, OAuth 2.0, and session authentication, you can ensure that your users are accessing a secure REST API. Similarly, authorization methods like role-based access control (RBAC), claims-based authorization, and policy-based authorization can also be implemented to ensure users only have access to the resources they are authorized for.
Step 4: Testing the API
When developing a Golang project, it is essential to have robust test coverage. Testing the REST API is no different. The process of testing a REST API can be broken down into two parts: setting up a test environment and writing unit and integration tests.
1. Setting up a Test Environment and Dependencies:
First, you must set up a test environment and install dependencies. This includes setting up tools such as Postman, a powerful tool for testing REST APIs. Other libraries such as mocks and assertions, are also important for writing comprehensive tests.
2. Writing Unit and Integration Tests:
Once the test environment is set up, you can start writing unit and integration tests for your Go Starter Kit Rest API. It is important to use TDD (Test Driven Development) methodology for writing these tests.
3. Using Tools Such as Postman to Test the API Endpoints:
When it comes to testing the API endpoints, Postman can be very useful. With Postman, you can easily create, send and review HTTP requests. You can also use the Postman collections feature to store the requests to use them in automated integration tests.
Testing a REST API requires time, effort, and knowledge of best practices. Using the right tools and having good test coverage is essential for ensuring that your Go Starter Kit Rest API works as expected.
The project structure also makes it easy to mock and inject the dependencies needed for testing, allowing you to focus on writing your tests instead of setting up complex fixtures. Additionally, there is an example Golang REST-API example on GitHub that you can use as a reference to ensure your code meets best practices.
Step 5: Deploying the API
Once your Golang REST API is ready to be deployed, you will need to consider a few things. Depending on your application, there may be specific requirements for deploying the API. Regardless of your specific requirements, there are a few steps to keep in mind when deploying a Golang REST API.
- If you are using a Go Starter Kit such as the golang clean architecture rest api or go starter kit available on GitHub, you can leverage the provided example code and configuration files to streamline the process. This step can save time and effort and make the process of deploying the API much simpler.
- It is important to consider the hosting environment when deploying your API. If you are using a cloud-based platform, such as Amazon Web Services or Google Cloud Platform, then you should use their provided tools and services to ensure that your API is securely hosted and able to scale as needed.
- After your API is up and running, you should ensure that all endpoints are working correctly and that security measures are in place. You may also want to consider testing your API with a service such as Postman or Insomnia to ensure it meets your application's requirements.
Once all of these steps are complete, your Golang REST API will be ready for use! By following these guidelines, you can ensure that your API is secure and reliable for use in production applications.
Step 6: Logging
Logging is a crucial component of any Golang project structure, especially when it comes to Rest APIs. Logging helps developers keep track of errors and other activities during development and debugging. For instance, by logging errors, developers can identify and address problems quickly.
Using a reliable logging solution can make the process of debugging a Go starter kit easier. Common logging solutions include Datadog, Sentry, and Elasticsearch. With Datadog, developers can quickly diagnose and debug issues in their Rest API applications. Sentry provides a powerful monitoring system to help catch errors and send notifications to developers. Finally, Elasticsearch enables developers to easily store, search, and analyze logs.
Using a reliable logging solution in your Golang Rest API starter kit can improve your applications' overall performance.
Suggested Read: Golang vs Java for Microservices
Conclusion
The Golang Rest API starter kit is a great way to quickly start your own API project. It comes with all the necessary components to swiftly scaffold a rest API, including setting up a database, creating handlers, and testing the API. With the help of the Golang Rest API starter kit, you can create a robust and efficient API in no time.
FAQs
1. How do I get started with creating a REST API in Go?
Once Golang is set up, it’s time to create your workspace. Next, you will need to write the code for your first endpoint. Once you’ve written your code, it’s time to test it out. You can use a tool such as Postman to send requests to your API endpoints and ensure they function correctly. Once you’ve tested your first endpoint, you can move on to adding more endpoints.
2. How do I structure my Go code for a REST API?
By separating the components into their own packages, you can easily identify where any changes need to be made and make sure they don't affect other parts of the application.
Also, it's important to use best practices such as using comments and documentation where appropriate, following standards for indentation, and separating business logic from view logic. This will help you find any potential errors or areas that need improvement.
3. How do I handle database interactions in my Go REST API?
While the native ‘database/SQL package is great for developers who are comfortable with SQL, those who are not may prefer an ORM library like GORM or working with a NoSQL database such as MongoDB or Redis. Whichever route you take, ensuring that your code is well-structured and tested will be key to creating a robust and secure REST API in Go.
4. How do I handle user authentication and authorization in my Go REST API?
The first option is to use a third-party library such as go-auth or oauth2. These libraries offer comprehensive solutions for user authentication and authorization. The second option is to build your own authentication system from scratch. This allows you to customize the system for your particular needs. To do this, you'll need to implement a session store, a way to encrypt passwords, and a way to limit access to certain resources.
Give your product vision the talent it deserves
building your dream engineering team.